Structures
array 배열과 structure 구조체의 차이는?
array는 동질적인 type의 데이터를 묶어서 보여주기 위해 사용하는 것이라면,
structure는 여러 타입의 변수를 통합하여 보여주기 위해 사용한다.
struct랑 typedef가 자주 묶여서 사용된다.
아래는 그 예시들
struct card{
int pips;
char suit;
}; typedef struct card card;
card c1, c2;
card c1, c2로 선언한 아이들의 pips, suit(멤버)에 접근하려면,
c1.pips, c2.suit와같이 .을 이용하면 된다.
멤버의 네임은 달라야 한다.
struct fruit {
char *name;
int calories;
}; typedef struct fruit fruit;
struct vegetable {
char *name;
int calories;
}; typedef struct vegetable vegetable;
fruit apple;
vegetable vet;
apple.calories = 100;
vet.calories = 120;
Accessing Members of a structure
#include <stdio.h>
#define CLASS_SIZE 100
struct student {
char *last_name;
int student_id;
char grade;
};
int fail(struct student class[]){
int i, cnt = 0;
for (i=0;i<CLASS_SIZE;i++){
cnt += class[i].grade == 'F';
}
return cnt;
}
int main(void){
struct student tmp, class[CLASS_SIZE];
tmp.grade = 'A';
tmp.last_name = "Yong";
tmp.student_id = 1111;
for (int i=0;i<CLASS_SIZE;i++){
class[i] = tmp;
}
printf("%d\n", fail(class));
return 0;
}
struct_pointer -> member name으로 멤버에 접근한다.
(포인터인 경우 -> 값인 경우 .)
(좌에서 우로 할당함)
struct complex {
double re;
double im;
}; typedef struct complex complex;
void add(complex *a, complex *b, complex *c){
a->re = b->re + c->re;
a->im = b->im + c->im;
}
.
#include <stdio.h>
struct student {
int id;
char* name;
char grade;
}; typedef struct student student;
int main(void){
student amy, zoo, young;
//값으로 접근
amy.id = 1;
amy.name = "amy";
amy.grade = 'A';
zoo.id = 2;
zoo.name = "Zoo";
zoo.grade = 'f';
young.id = 3;
young.name = "Young";
young.grade = 'b';
// 포인터로 접근
student *p = &amy, *q = &zoo, *r = &young;
printf("%d\n%d\n%d\n", p->id, q->id, r->id);
return 0;
}
using structures with functions
employee data - 수정 필요함
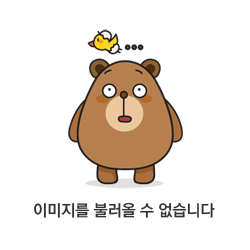
initialization of structures
struct card {
int pips;
char suit;
};
typedef struct card card;
card c = {13,'h'};
typedef struct {
float re;
float im;
} complex;
complex a[3][3] = {
{{1.0, -0.1}, {2.0, 0.2}, {3.0, 0.3}},
{{4.0,-0.4}, {5.0, 0.5}, {6.0, 0.0}}
};
unions 공용체
struct와 유사하지만, union 변수 중 가장 큰 변수의 메모리를 모든 변수가 공유한다.
가장 큰 변수를 기준으로 메모리를 할당한다.
통신에서 아주 편리하게 사용됨
union int_or_float {
int i;
float f;
};
union int_or_float a, b, c;
비트 활용에서 구조체 사용..?
'Computer Science > C' 카테고리의 다른 글
C언어 7강 | Bitwise operators (0) | 2021.12.17 |
---|---|
C언어 10강 | Structures and list processing | 제일 중요함 *** (0) | 2021.12.17 |
C언어 6강 | Arrays, Pointers, and Strings | 중요! (0) | 2021.12.16 |
C언어 4강 | Flow of Control | 반복문, 조건문, 순차 구조 (0) | 2021.12.16 |
C언어 3강 | 데이터 타입 | char, int(short, long,unsigned), float, typedef (0) | 2021.12.16 |